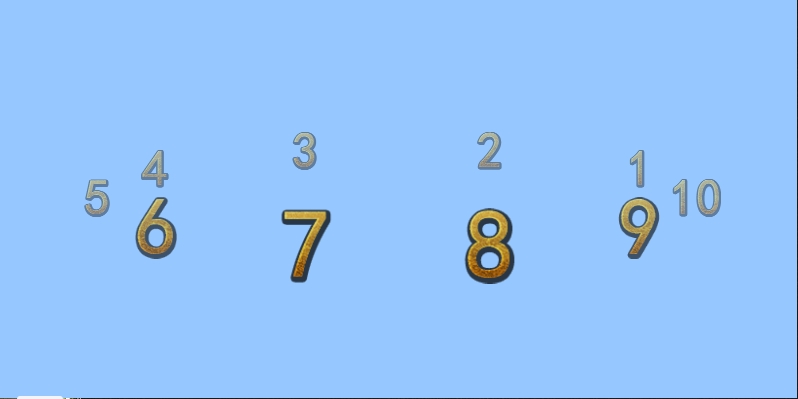
local container = GUI:Layout_Create(ImageView, "container", 0, 54.00, 800.00, 400.00, false)
GUI:Layout_setBackGroundColorType(container, 1)
GUI:Layout_setBackGroundColor(container, "#96c8ff")
local containerSize = GUI:getContentSize(container)
local centerX, centerY = containerSize.width / 2, containerSize.height / 2
local a, b = 300, 100 -- 椭圆的长轴和短轴
local viewCount = 10
local images = {}
local nowRadian = {}
local touchStartX = 0
local isTouching = false
-- 计算椭圆各个控件的落点坐标
local function getEllipsePointAt(radian)
return {
x = a * math.cos(radian) + centerX,
y = b * math.sin(radian) + centerY,
}
end
-- 计算权重
local function getWeight(y)
local weight = 1 - (y - (centerY - b)) / (2 * b)
if weight < 0.5 then
weight = 0.5
elseif weight > 1 then
weight = 1
end
return weight
end
-- 更新视图
local function updateView()
for i = 1, viewCount do
local point = getEllipsePointAt(nowRadian[i])
local image = images[i]
GUI:setPosition(image, point.x, point.y)
local weight = getWeight(point.y)
GUI:setScale(image, weight)
GUI:setOpacity(image, 255 * weight)
GUI:setLocalZOrder(image, math.floor(weight * 10))
end
end
-- 初始化界面
local function initView()
for i = 1, viewCount do
local radian = (math.pi * 2) / viewCount * i
local point = getEllipsePointAt(radian)
local image = GUI:Image_Create(container, "img" .. i, point.x, point.y, string.format("res/public/word_fubentg_%d.png", i))
GUI:setAnchorPoint(image, 0.5, 0.5)
nowRadian[i] = radian
images[i] = image
local weight = getWeight(point.y)
GUI:setScale(image, weight)
GUI:setOpacity(image, 255 * weight)
GUI:setLocalZOrder(image, math.floor(weight * 10))
end
end
-- 处理触摸事件
local function onTouchBegan(x)
touchStartX = x
isTouching = true
end
local function onTouchMoved(x)
if isTouching then
local deltaX = x - touchStartX
touchStartX = x
local angleChange = deltaX / 100 -- 调整滑动速度
for i = 1, viewCount do
nowRadian[i] = nowRadian[i] + angleChange
end
updateView()
end
end
local function onTouchEnded()
isTouching = false
end
-- 注册触摸事件
GUI:setTouchEnabled(container, true)
GUI:addOnTouchEvent(container, function(sender, type)
if type == 0 then -- 触摸开始
local location = GUI:getTouchBeganPosition(sender)
onTouchBegan(location.x)
elseif type == 1 then -- 触摸移动
local location = GUI:getTouchMovePosition(sender)
onTouchMoved(location.x)
elseif type == 2 or type == 3 then -- 触摸结束或取消
local location = GUI:getTouchEndPosition(sender)
onTouchEnded()
end
end)
-- 调用初始化函数
initView()
|